REST vs SOAP: why we recommend REST APIs for A2P messaging
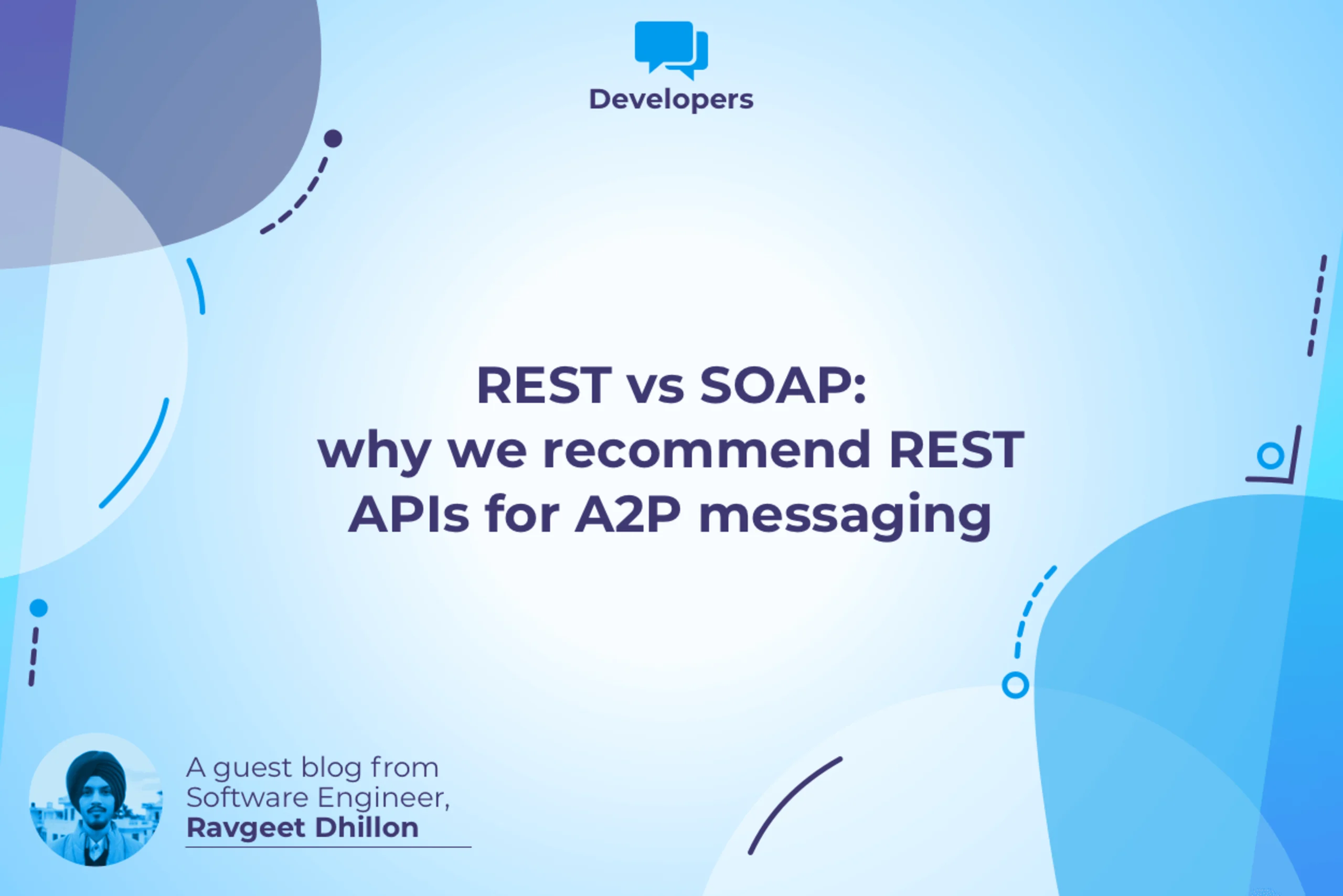
By Guest Post
December 12, 2023
5 minutes
This blog was originally posted on September 30, 2022
- developers
- rest api
- sms api
- apis
- soap api
- messaging apps
By Guest Post
December 12, 2023
5 minutes