Build SMS autoresponders in Python that work like magic
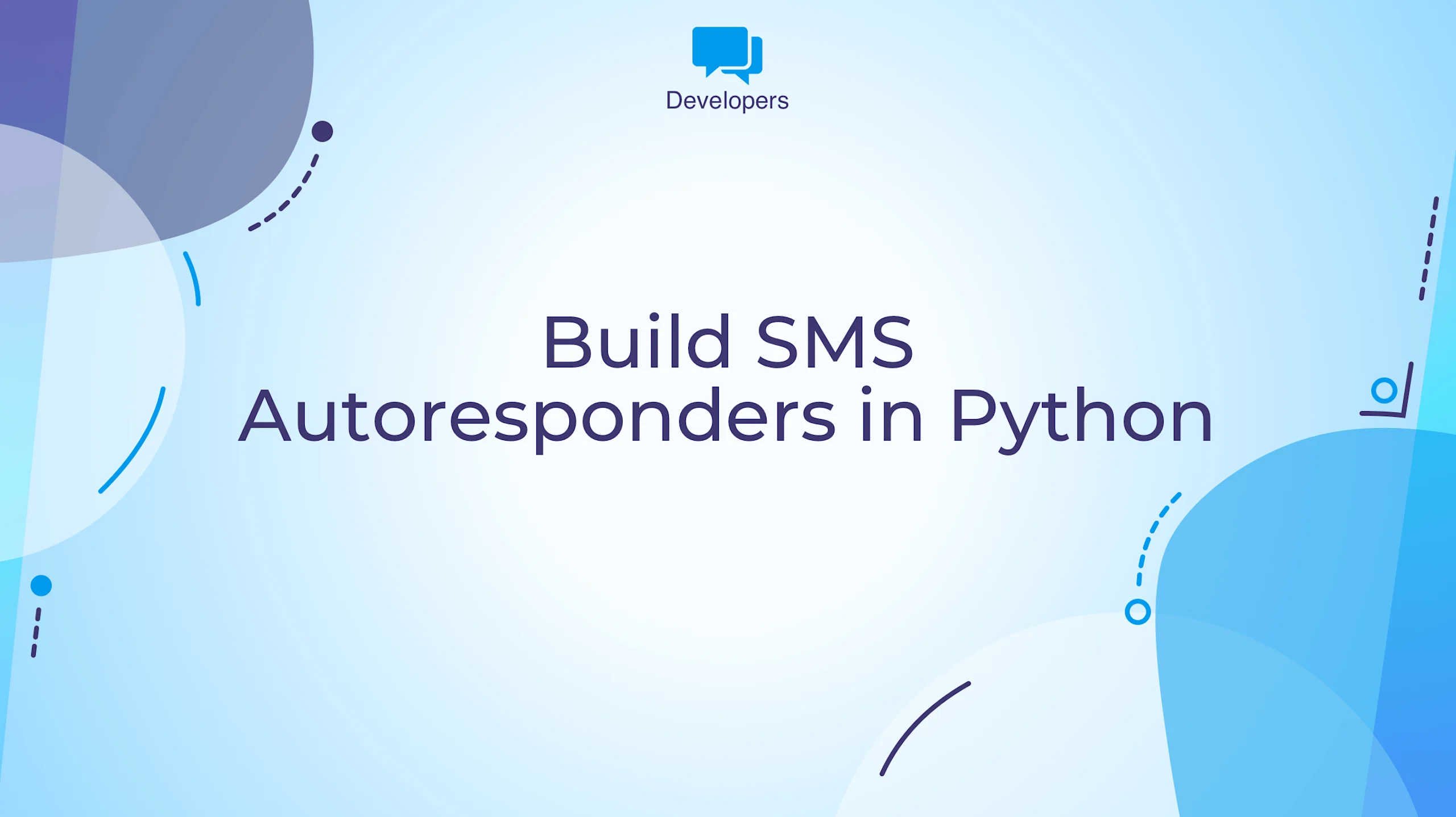
By Team ClickSend
October 24, 2023
6 minutes
This blog was originally posted on March 30, 2023
- developers
- sms autoresponder
- send sms in python
- python sms sdk
By Team ClickSend
October 24, 2023
6 minutes